Introduction
When using the Settings API you can define a custom field type by creating a function called settings_{your_custom_field_type}. The text after the first underscore will be what you decided to name your type. The function will run for every field of that type.
Important: The Settings API is not used to create custom form fields. To create custom form fields you would need to extend the GF_Field class, part of the Field Framework.
Examples
The following example renders the field for the field type my_custom_field_type. This function creates two text box fields.:
public function plugin_settings_fields() {
return array(
array(
'title' => 'My Custom Field',
'description' => 'This is a description of the purpose of Section 1',
'fields' => array(
array(
'label' => 'My Custom Field',
'type' => 'my_custom_field_type',
'name' => 'my_custom_field',
)
)
),
);
}
public function settings_my_custom_field_type() {
$this->settings_text(
array(
'label' => 'Item 1',
'name' => 'my_custom[1]',
'default_value' => 'Item 1'
)
);
$this->settings_text(
array(
'label' => 'Item 2',
'name' => 'my_custom[2]',
'default_value' => 'Item 2'
)
);
}
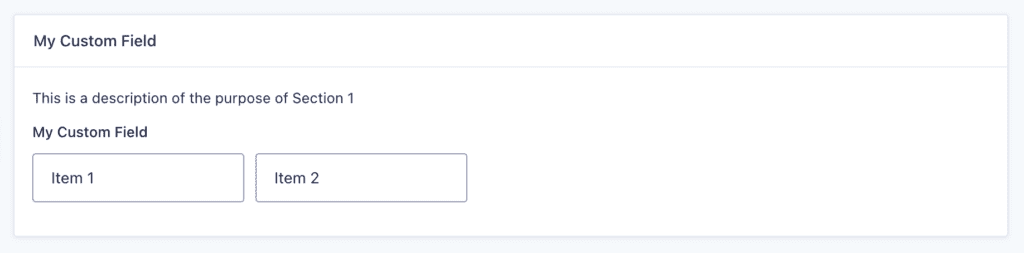
The following example has a custom field type named “text_with_checkbox”. This custom type creates a text box field and a checkbox field.
public function plugin_settings_fields() {
return array(
array(
'title' => 'Custom Text with Checkbox',
'description' => 'This is a description of the purpose of Section 1',
'fields' => array(
array(
'label' => 'Text box with a checkbox',
'type' => 'text_with_checkbox',
'name' => 'text_checkbox',
)
)
),
);
}
// This is a custom field type which will create a text box and a check box
public function settings_text_with_checkbox() {
$this->settings_text(
array(
'label' => 'Text Box',
'name' => 'txt_cbx[1]',
'default_value' => 'Item 1'
)
);
$this->settings_checkbox(
array(
'label' => 'Checkbox',
'choices' => array(
array(
'label' => 'Checkbox',
'name' => 'txt_cbx[2]'
)
)
)
);
}
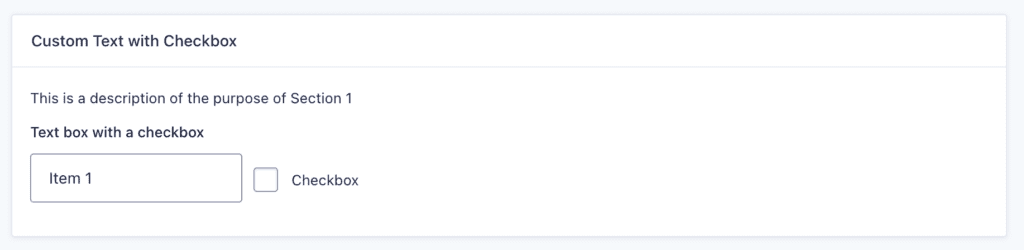