Description
This filter allows you to override the default choice markup used when rendering radio button, checkbox and drop down type fields.
Usage
To apply the filter to all radio, checkbox and drop down type fields on all forms you can do this:
add_filter( 'gform_field_choice_markup_pre_render', 'your_function_name', 10, 4 );
To target a specific form append the form id to the hook name. (format: gform_field_choice_markup_pre_render_FORMID)
add_filter( 'gform_field_choice_markup_pre_render_10', 'your_function_name', 10, 4 );
To target a specific field on a specific form append the form id and the field id to the hook name. (format: gform_field_choice_markup_pre_render_FORMID_FIELDID)
add_filter( 'gform_field_choice_markup_pre_render_10_5', 'your_function_name', 10, 4 );
Parameters
- $choice_markup string
The string containing the choice markup to be filtered.
Checkbox
<li class='gchoice_10_5_1'>
<input name='input_5.1' type='checkbox' value='Item 1|10' id='choice_10_5_1' tabindex='1' />
<label for='choice_10_5_1' id='label_10_5_1'>First Choice</label>
</li>
Radio
<li class='gchoice_10_5_0'>
<input name='input_5' type='radio' value='Item 1|10' id='choice_10_5_0' tabindex='1' />
<label for='choice_10_5_0' id='label_10_5_0'>First Choice</label>
</li>
Select
<option value='Item 1' selected='selected'>First Choice</option>
- $choice array
An associative array containing the choice properties.
array(
'text' => 'First Choice',
'value' => 'Item 1',
'isSelected' => true,
'price' => '10',
)
- $field Field Object
The field currently being processed. - $value string
The value to be selected if the field is being populated.
Examples
1. Add Custom Attribute
This example demonstrates how to add a custom attribute to the choice markup.
add_filter( 'gform_field_choice_markup_pre_render_10', function ( $choice_markup, $choice, $field, $value ) {
if ( $field->get_input_type() == 'radio' && rgar( $choice, 'text' ) == 'Second Choice' ) {
return str_replace( "type='radio'", "type='radio' disabled", $choice_markup );
}
return $choice_markup;
}, 10, 4 );
2. Include Price for Product Fields
This example shows how you can update the option markup to include the choice price for the drop down type Product field.
add_filter( 'gform_field_choice_markup_pre_render', function ( $choice_markup, $choice, $field, $value ) {
if ( $field->type == 'product' ) {
$new_string = sprintf( '>%s - %s<', $choice['text'], GFCommon::to_money( $choice['price'] ) );
return str_replace( ">{$choice['text']}<", $new_string, $choice_markup );
}
return $choice_markup;
}, 10, 4 );
3. Remove choice based on date
This example shows how you can remove a choice from a specific field if the specified date has passed.
add_filter( 'gform_field_choice_markup_pre_render_10_5', function ( $choice_markup, $choice ) {
if ( rgar( $choice, 'value' ) == 'test' && time() >= strtotime( '2019-10-03' ) ) {
return '';
}
return $choice_markup;
}, 10, 2 );
4. Add CSS class to Multiselect choice
This example shows how you can add a CSS class to a Multiselect field choice labeled as “Second Choice”.
// Change 543 and 2 to your form id and field id respectively.
add_filter( 'gform_field_choice_markup_pre_render_543_2', function ( $choice_markup, $choice, $field, $value ) {
// Change Second Choice to the label of your choice.
if ( $field->get_input_type() == 'multiselect' && rgar( $choice, 'text' ) == 'Second Choice' ) {
// Change my_class to the CSS class name you want to use.
return str_replace( "value=", "class='my_class' value=", $choice_markup );
}
return $choice_markup;
}, 10, 4 );
5. Add inline list headings
This example shows how you can replace a choice with an inline heading, by prepending your option label with ###.
add_filter( 'gform_field_choice_markup_pre_render', function( $choice_markup, $choice, $field, $value ) {
if ( strpos( $choice['text'], '###' ) === 0 ) {
$choice_markup = '<li><span>' . ltrim( str_replace( '###', '', $choice['text'] ) ) . '</span></li>';
}
return $choice_markup;
}, 10, 4 );
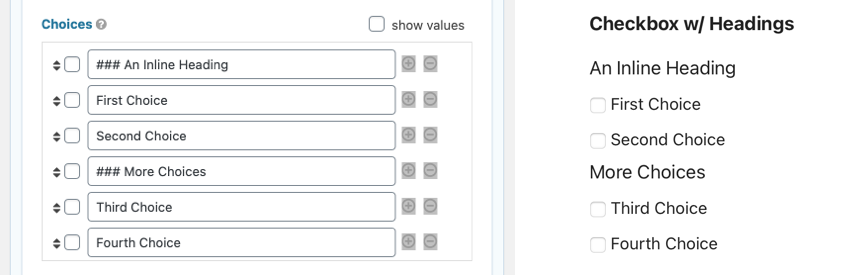
Placement
This code should be placed in the functions.php file of your active theme.
Source Code
This filter is located in the following methods:
- GFCommon::get_select_choices() in common.php – since 1.9.11.10
- GF_Field_Checkbox::get_checkbox_choices() in includes/fields/class-gf-field-checkbox.php – since 1.9.6
- GF_Field_Radio::get_radio_choices() in includes/fields/class-gf-field-radio.php – since 1.9.6