Disclaimer
Gravity Forms does not officially support nested conditional logic. This means a field should not have conditional logic based on a field that also has conditional logic. Some clever folks have come up with workarounds or specific scenarios they feel work, but these are not officially supported by us.
With that in mind, here is some info that may or may not be of use!
When will nested conditional logic work?
Nested conditional logic will work if the conditional field does not have a default value, is not dynamically populated, and, if the field is a Drop Down, it uses a placeholder.
When will nested conditional logic not work?
Nested conditional logic will not work if:
- the conditional field has a default value
- the conditional field is dynamically populated
- if the conditional field is a Drop Down that does not use a placeholder
- the values of earlier fields are changed on multipage or conversational forms
When Gravity Forms hides a field via conditional logic, it resets the field’s initial value. Conditional logic dependent on this field will be evaluated with that reset value rather than an empty value, which is unexpected.
Workarounds
Possible Approach
Note: While adding extra conditions to account for nested dependencies can help, this approach suits simpler logic requirements and may not fully resolve issues for users dealing with complex nested conditions.
The best method to support nested conditional logic is to add additional conditions to account for the nested dependency.
For example:
- Field B is conditionally dependent upon Field A
- you want Field C to be conditionally dependent upon Field B
In this case, you would set up Field C’s dependence on B, but also add the conditions that you configured for Field B onto Field C as well. In this way, you make Field C explicitly dependent on both Field B and Field A.
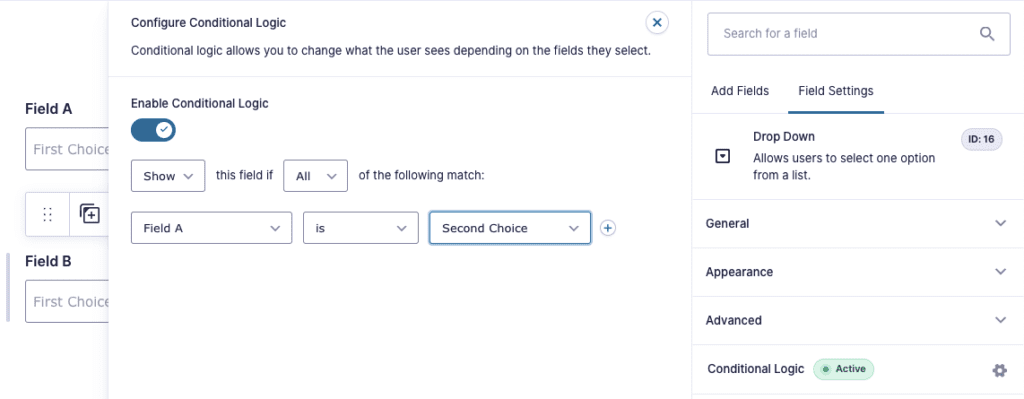
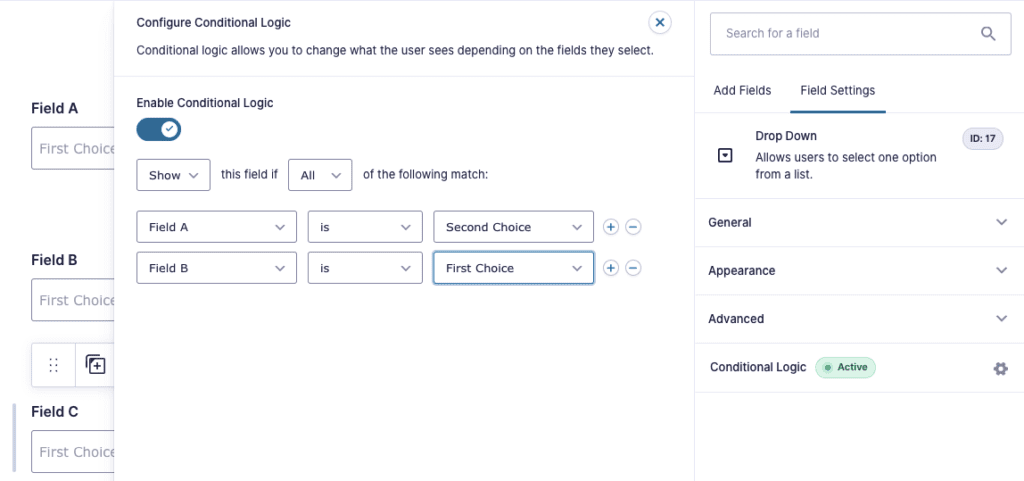
Drop Down Placeholders
Nested conditional logic issues are most commonly exposed by Drop Downs. If the Drop Down does not have a choice with an empty value (such as a placeholder), the Drop Down’s value will be reset to that of the Drop Down’s first option when the field is hidden via conditional logic. Since the field is hidden, many users expect the value of the field to be empty and create nested conditional logic rules based on this assumption.
The simplest solution is to make sure your Drop Down has a placeholder. In this way, the Drop Down value will be reset to an empty value (via the placeholder) when hidden via conditional logic. Note: This does not address the issues with setting a default value and dynamic population mentioned above.
Finding nested conditional Logic
To check if your form has any nested conditional logic, copy and paste this script into your browser’s console on the form editor page.
var deps = {};
for( var i = 0; i < form.fields.length; i++ ) {
var field = form.fields[i];
if( ! field.conditionalLogic ) { continue; }
if( typeof deps[ field.id ] === 'undefined' ) { deps[ field.id ] = []; }
for( var j = 0; j < field.conditionalLogic.rules.length; j++ ) {
var rule = field.conditionalLogic.rules[j];
if( jQuery.inArray( rule.fieldId, deps[ field.id ] ) === -1 ) {
deps[ field.id ].push( rule.fieldId );
}
}
}
for( var fieldId in deps ) {
if( ! deps.hasOwnProperty( fieldId ) ) { continue; }
for( i = 0; i < deps[ fieldId ].length; i++ ) {
var depFieldId = deps[ fieldId ][i]; // Does current dependent field have any dependencies?
if( typeof deps[ depFieldId ] !== 'undefined' ) {
console.log( 'Nested dependency found', '|', 'ID:', fieldId, '|', 'Label:', GetFieldById( fieldId ).label );
}
}
}
It will output a message to the browser Console Logs for each dependency found.
Nested dependency found | ID: 3 | Label: Field C