Introduction
Connecting WooCommerce product data to Gravity Forms allows you to fill form fields with product details, such as titles and featured images.
This article demonstrates using a custom snippet to retrieve WooCommerce products and populate a Gravity Forms Image Choice field.
Example One
This example shows how to populate products using the featured image as the image in the Image Choice field.
add_filter( 'gform_pre_render', 'populate_image_choices' );
add_filter( 'gform_pre_validation', 'populate_image_choices' );
add_filter( 'gform_admin_pre_render', 'populate_image_choices' );
add_filter( 'gform_pre_submission_filter', 'populate_image_choices' );
function populate_image_choices( $form ) {
if ( rgar( $form, 'id' ) != 123 ) { // replace 123 with your form id
return $form;
}
$posts = get_posts( array(
'post_type' => 'product',
'post_status' => 'publish',
'posts_per_page' => -1,
));
$items = array();
foreach ( $posts as $post ) {
$product = wc_get_product( $post->ID );
if ( !$product ) {
continue;
}
$image_id = $product->get_image_id();
$image_url = wp_get_attachment_url( $image_id );
if ( $image_url ) {
$items[] = array(
'text' => $product->get_name(),
'value' => $product->get_name(),
'image' => $image_url,
'file_url' => $image_url,
'attachment_id' => $image_id
);
}
}
foreach ( $form['fields'] as &$field ) {
if ( $field->id == 1 ) { // Replace 1 with your image choice field id
$field->choices = $items;
break;
}
}
return $form;
}
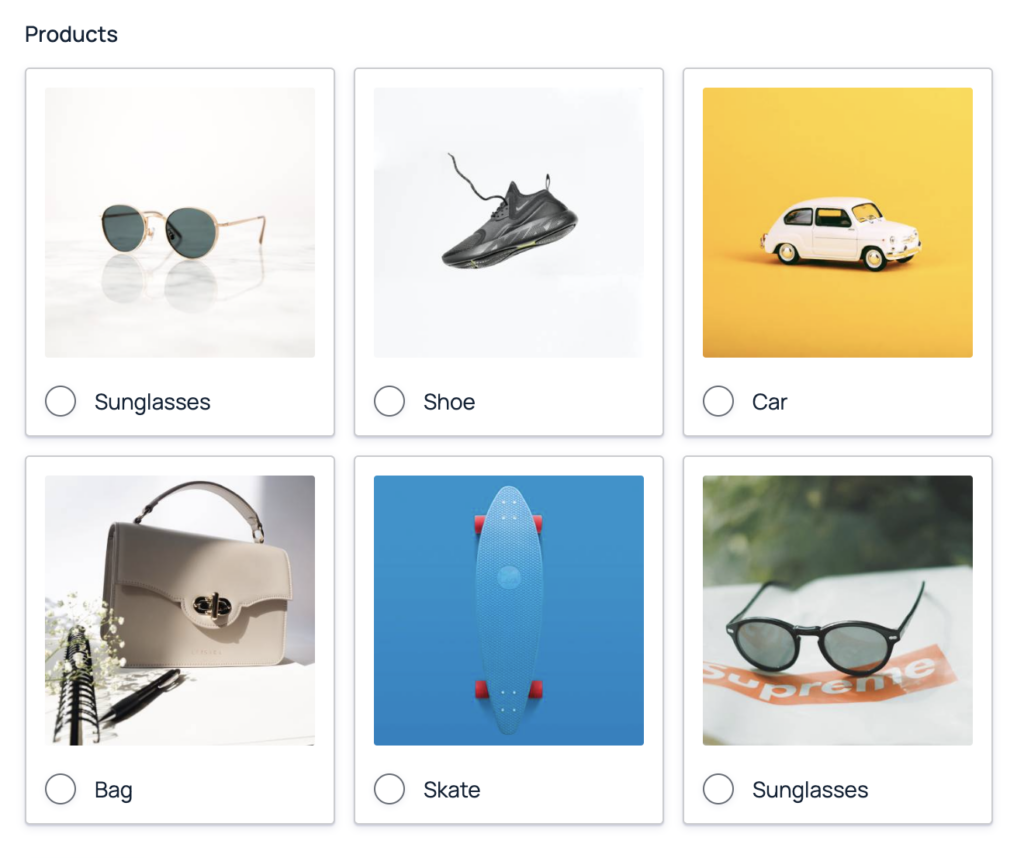
Example Two
This example shows how to populate products using the featured image as the image in the Image Choice field, including the Price.
add_filter( 'gform_pre_render', 'populate_image_choices' );
add_filter( 'gform_pre_validation', 'populate_image_choices' );
add_filter( 'gform_admin_pre_render', 'populate_image_choices' );
add_filter( 'gform_pre_submission_filter', 'populate_image_choices' );
function populate_image_choices( $form ) {
if ( rgar( $form, 'id' ) != 535 ) {
return $form;
}
$posts = get_posts( array(
'post_type' => 'product',
'post_status' => 'publish',
'posts_per_page' => 6,
));
$items = array();
foreach ( $posts as $post ) {
$product = wc_get_product( $post->ID );
if ( !$product ) {
continue;
}
$image_id = $product->get_image_id();
$image_url = wp_get_attachment_url( $image_id );
if ( $image_url ) {
// Append price to product name
$price = wc_format_decimal( $product->get_price(), 2 ); // Ensures proper decimal formatting
$name_with_price = $product->get_name() . ' (' . wc_price( $price ) . ')';
$items[] = array(
'text' => $name_with_price,
'value' => $product->get_id(), // Use product ID as value
'image' => $image_url,
'file_url' => $image_url,
'attachment_id' => $image_id
);
}
}
foreach ( $form['fields'] as &$field ) {
if ( $field->id == 1 ) {
$field->choices = $items;
break;
}
}
return $form;
}
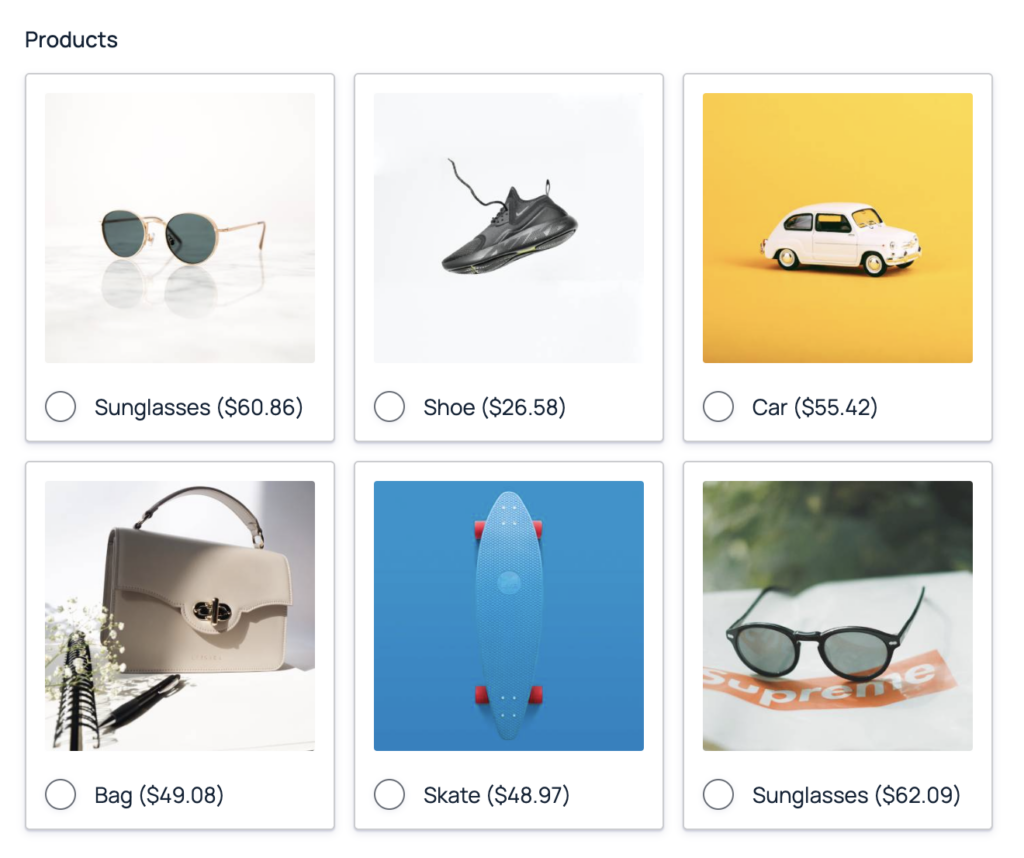
Example Three
This snippet integrates Gravity Forms with WooCommerce by automatically adding a selected product to the WooCommerce Cart.
add_action('gform_after_submission_123', function($entry) {
$product_id = rgar($entry, '1'); // Ensure this matches your actual field ID
if (!$product_id) {
return;
}
$product = wc_get_product($product_id);
if ($product) {
WC()->cart->empty_cart(); // Optional: Clear previous cart items
WC()->cart->add_to_cart($product_id, 1); // Add selected product
// Simulate WooCommerce Add to Cart action
do_action('woocommerce_add_to_cart', $product_id);
}
}, 10, 2);
You can redirect the user to the checkout page after submitting the form with a custom Confirmation page.
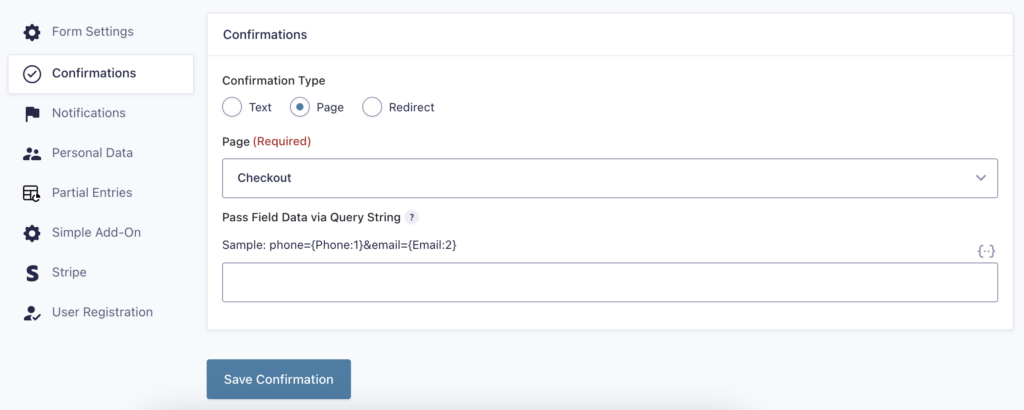